Key Data Annotation Auto Generated
- Jan 15, 2016 Key Attribute. You can override this behaviour using the Data Annotation Key attribute. Entity Framework Data Annotation Key attribute marks the property as Primary Key. This will override the default Primary Key. The following Code creates the table with CustomerNo as the Primary key instead if CustomerID. Do not forget to import the following.
- What I want to do is to create a primary key that has auto decrement: Id BIGINT PRIMARY KEY IDENTITY(-1,-1) I searched for it and I could only find the following DataAnnotation for setting the Identity: DatabaseGenerated(DatabaseGeneratedOption.Identity) But this not fullfill my need of setting start and increment values.
- Before creating the model, Code First takes convention into consideration first, then reads the data annotation attributes and finally reads the mappings specified by Fluent API. The order of priorities for creating primary key using Entity Framework Code First is: Convention - Data Annotation - Fluent API (Highest Priority).
May 28, 2013 Hibernate provides the facility to update the entity for those database values which are generated at insert or row update time. @Generated belongs to org.hibernate.annotations. @Generated is used at property level. There are different GenerationTime. GenerationTime.INSERT GenerationTime.INSERT updates the entity at insert time. Data Annotations - Key Attribute in EF 6 & EF Core. The Key attribute can be applied to a property in an entity class to make it a key property and the corresponding column to a PrimaryKey column in the database. The default convention creates a primary key column for a property whose name is Id or Id. The Key attribute.
Value generation patterns
There are three value generation patterns that can be used for properties:
- No value generation
- Value generated on add
- Value generated on add or update
No value generation
No value generation means that you will always supply a valid value to be saved to the database. This valid value must be assigned to new entities before they are added to the context.
Value generated on add
Value generated on add means that a value is generated for new entities.
Depending on the database provider being used, values may be generated client side by EF or in the database. If the value is generated by the database, then EF may assign a temporary value when you add the entity to the context. This temporary value will then be replaced by the database generated value during SaveChanges()
.
If you add an entity to the context that has a value assigned to the property, then EF will attempt to insert that value rather than generating a new one. A property is considered to have a value assigned if it is not assigned the CLR default value (null
for string
, 0
for int
, Guid.Empty
for Guid
, etc.). For more information, see Explicit values for generated properties.
Warning
How the value is generated for added entities will depend on the database provider being used. Database providers may automatically setup value generation for some property types, but others may require you to manually setup how the value is generated.
For example, when using SQL Server, values will be automatically generated for GUID
properties (using the SQL Server sequential GUID algorithm). However, if you specify that a DateTime
property is generated on add, then you must setup a way for the values to be generated. One way to do this, is to configure a default value of GETDATE()
, see Default Values.
Value generated on add or update
Value generated on add or update means that a new value is generated every time the record is saved (insert or update).
Like value generated on add
, if you specify a value for the property on a newly added instance of an entity, that value will be inserted rather than a value being generated. It is also possible to set an explicit value when updating. For more information, see Explicit values for generated properties.
Warning
How the value is generated for added and updated entities will depend on the database provider being used. Database providers may automatically setup value generation for some property types, while others will require you to manually setup how the value is generated.
For example, when using SQL Server, byte[]
properties that are set as generated on add or update and marked as concurrency tokens, will be setup with the rowversion
data type - so that values will be generated in the database. However, if you specify that a DateTime
property is generated on add or update, then you must setup a way for the values to be generated. One way to do this, is to configure a default value of GETDATE()
(see Default Values) to generate values for new rows. You could then use a database trigger to generate values during updates (such as the following example trigger).
Value generated on add
By convention, non-composite primary keys of type short, int, long, or Guid are set up to have values generated for inserted entities, if a value isn't provided by the application. Your database provider typically takes care of the necessary configuration; for example, a numeric primary key in SQL Server is automatically set up to be an IDENTITY column.
You can configure any property to have its value generated for inserted entities as follows:
Warning
This just lets EF know that values are generated for added entities, it does not guarantee that EF will setup the actual mechanism to generate values. See Value generated on add section for more details.
Default values
On relational databases, a column can be configured with a default value; if a row is inserted without a value for that column, the default value will be used.
You can configure a default value on a property:
You can also specify a SQL fragment that is used to calculate the default value:
Specifying a default value will implicitly configure the property as value generated on add.
Value generated on add or update
Warning
This just lets EF know that values are generated for added or updated entities, it does not guarantee that EF will setup the actual mechanism to generate values. See Value generated on add or update section for more details.
Computed columns
On some relational databases, a column can be configured to have its value computed in the database, typically with an expression referring to other columns:
Note
In some cases the column's value is computed every time it is fetched (sometimes called virtual columns), and in others it is computed on every update of the row and stored (sometimes called stored or persisted columns). This varies across database providers.
No value generation
Disabling value generation on a property is typically necessary if a convention configures it for value generation. For example, if you have a primary key of type int, it will be implicitly set configured as value generated on add; you can disable this via the following:
Primary Key Generation Using Oracle's Sequence
Oracle provides the sequence
Cengage mindtap access code key generator. utility to automatically generate unique primary keys. To use this utility to auto-generate primary keys for a CMP entity bean, you must create a sequence table and use the @AutomaticKeyGeneration annotation to point to this table.
In your Oracle database, you must create a sequence table that will create the primary keys, as shown in the following example:
This creates a sequences of primary key values, starting with 1, followed by 2, 3, and so forth. The sequence table in the example uses the default increment 1, but you can change this by specifying the increment keyword, such as increment by 3. When you do the latter, you must specify the exact same value in the cacheSize attribute of the @AutomaticKeyGeneration annotation:
If you have specified automatic table creation in the CMP bean's project settings, the sequence table will be created automatically when the entity bean is deployed. For more information, see @JarSettings Annotation. For more information on the definition of a CMP entity bean, see below.
Primary Key Generation Using SQL Server's IDENTITY
In SQL Server you can use the IDENTITY
keyword to indicate that a primary-key needs to be auto-generated. The following example shows a common scenario where the first primary key value is 1, and the increment is 1:
In the CMP entity bean definition you need to specify SQLServer(2000) as the type of automatic key generator you are using. You can also provide a cache size:
If you have specified automatic table creation in the CMP bean's project settings, the sequence table will be created automatically when the entity bean is deployed. For more information, see @JarSettings Annotation. For more information on the definition of a CMP entity bean, see below.
Primary Key Generation Using a Named Sequence Table
A named sequence table is similar to the Oracle sequence functionality in that a dedicated table is used to generate primary keys. However, the named sequence table approach is vendor-neutral. To auto-generate primary keys this way, create a named sequence table using the two SQL statements shown in the example:
In the CMP entity bean definition you need to specify the named sequence table as the type of automatic key generator you are using. You can also provide a cache size:
If you have specified automatic table creation in the CMP bean's project settings, the sequence table will be created automatically when the entity bean is deployed. For more information, see @JarSettings Annotation. For more information on the definition of a CMP entity bean, see the next section.
Note. When you specify a cacheSize value for a named sequence table, a series of unique values are reserved for entity bean creation. When a new cache is necessary, a second series of unique values is reserved, under the assumption that the first series of unique values was entirely used. This guarantees that primary key values are always unique, although it leaves open the possibility that primary key values are not necessarily sequential. For instance, when the first series of values is 10..20, the second series of values is 21-30, even if not all values in the first series were actually used to create entity beans.
Defining the CMP Entity Bean
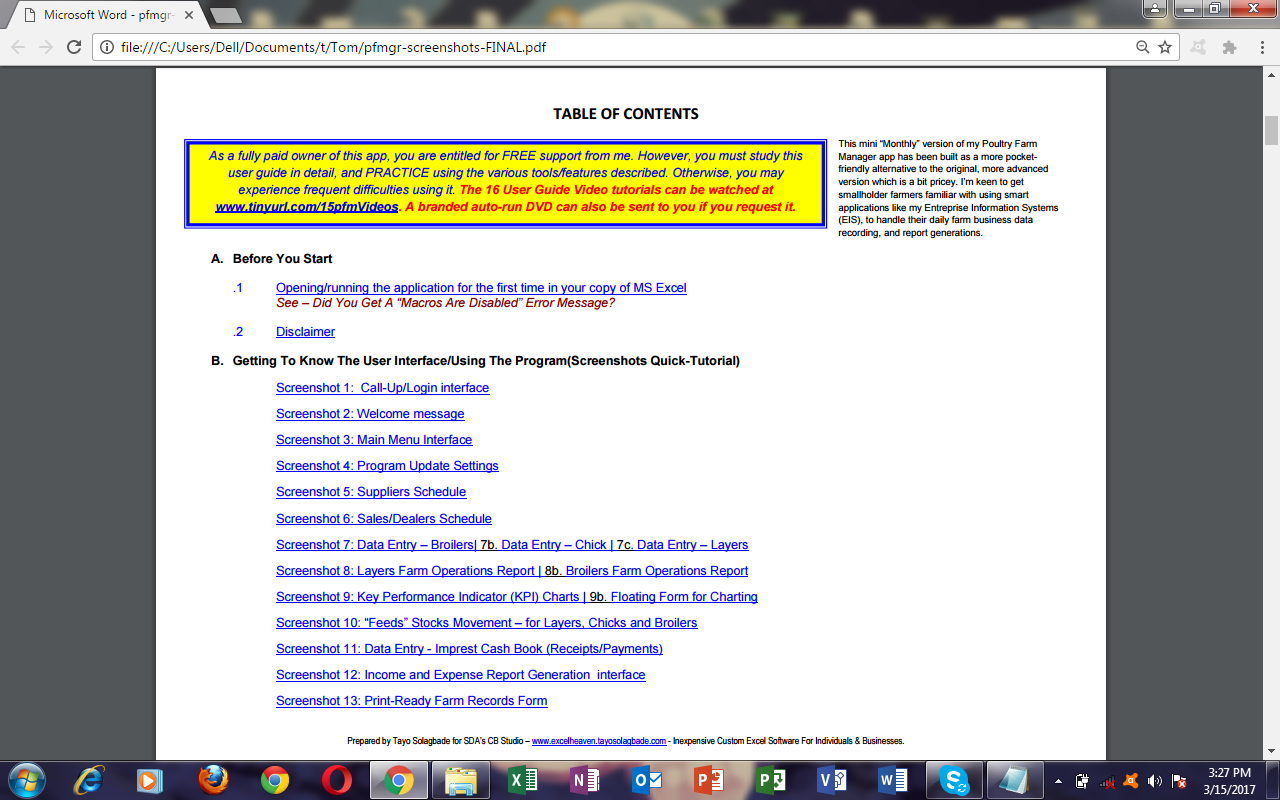
When defining a CMP entity bean that uses one of the primary key generators, you use the the @AutomaticKeyGeneration annotation to point to the name of the primary key generator table to obtain primary keys. Also, you must define a primary key field of type Integer or Long to set and get the auto-generated primary key. However, the ejbCreate method does not take a primary key value as an argument. Instead the EJB container adds the correct primary key to the entity bean record.
Key Data Annotation Auto Generated Free
The following example shows what the entity bean might look like. Notice that the bean uses the named sequence option described above, and that ejbCreate method does not take a primary key:Data Annotation Definition
Related Topics